Themes
Learn how to customize the themes in your MkSaaS website
This guide covers the theme system in your MkSaaS website, how to switch between built-in themes, and how to create and customize your own themes.
Core Features
The MkSaaS template comes with a built-in theme system that allows users to customize the appearance of the website. The theme system includes:
- Light and dark mode support
- Multiple color themes (default, neutral, blue, green, amber)
- Theme switching functionality for users
- Theme persistence with cookies
Built-in Themes
The template includes several pre-configured themes that you can use out of the box.
Available Themes
The following themes are available by default:
- Default: The main theme with a balanced color palette
- Neutral: A grayscale theme with neutral colors
- Blue: A theme with blue as the primary color
- Green: A theme with green as the primary color
- Amber: A theme with amber as the primary color
Each theme changes the primary color and related colors while maintaining the overall design system.
Theme Configuration
The theme system is configured in the website.tsx
configuration file.
Configuration Options:
Property | Type | Description |
---|---|---|
defaultTheme | 'default' | 'blue' | 'green' | 'amber' | 'neutral' | Sets the default color theme for the website |
enableSwitch | boolean | When true, allows users to change the color theme |
How Theme Switching Works
The theme switching functionality is implemented through several key components:
1. Theme Provider
The ActiveThemeProvider
component in src/components/layout/active-theme-provider.tsx
manages the current theme state and persists it using cookies:
2. Theme Selector Component
The ThemeSelector
component in src/components/layout/theme-selector.tsx
provides the UI for users to switch between themes:
3. CSS Implementation
The themes are defined in the global CSS file (src/styles/globals.css
) using CSS variables and Tailwind CSS:
Creating Custom Themes
You can create your own custom themes by adding new theme definitions to the global CSS file.
1. Add a New Theme Class
Add a new theme class to src/styles/globals.css
:
2. Add the Theme to the Selector
Modify the ThemeSelector
component in src/components/layout/theme-selector.tsx
to include your new theme:
3. Add the Translation Key
Add a new translation key for your theme in your translation files.
Advanced Customization
For more advanced theme customization, you can modify the base theme variables.
Customizing the Base Theme
The base theme variables are defined in the :root
selector in src/styles/globals.css
. You can modify these variables to change the default appearance across all themes:
Creating a Custom Color Palette
You can create a completely custom color palette by overriding all color variables in your theme class:
Theme Generators
You can use the following theme generators to create your own custom themes, and then copy the CSS variables to your globals.css
file.
This screenshot shows the default theme is now the custom theme.
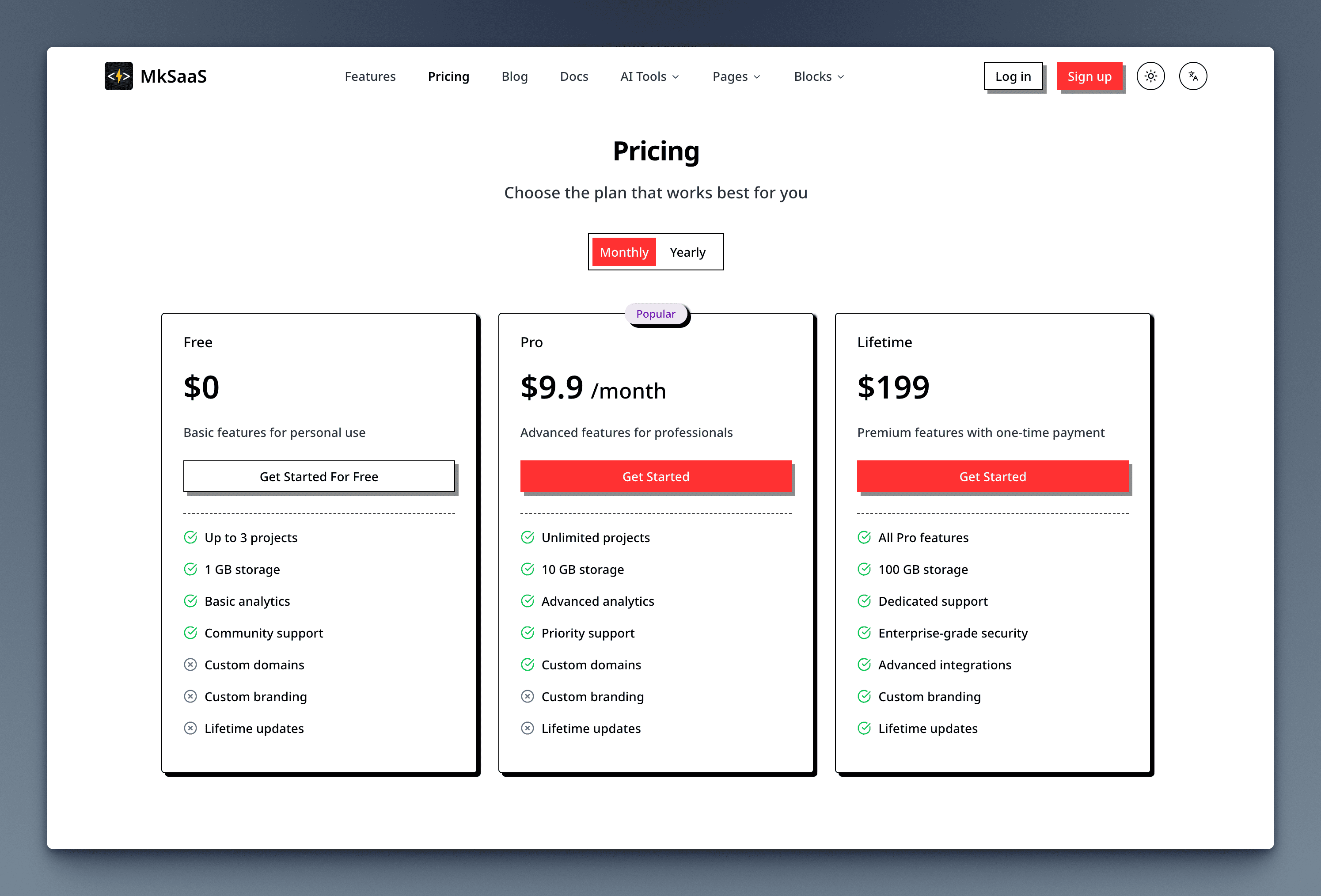
Troubleshooting
Theme Not Applying
If your theme is not applying correctly:
- Check that the theme class is correctly defined in
globals.css
- Verify that the theme selector is enabled in website configuration
- Check browser console for any JavaScript errors
- Clear browser cookies and reload the page
Custom Theme Flashing on Page Load
If you see a flash of the default theme before your custom theme loads:
- Set your custom theme as the default theme in
website.tsx
- Use a loading state or skeleton UI while the theme loads
- Consider implementing server-side rendering for the initial theme
For server-side rendering, modify your layout component to pass the initial theme from cookies to the ActiveThemeProvider
.
Best Practices
- Color Contrast: Ensure that text colors have sufficient contrast with background colors for accessibility
- Test Both Modes: Always test your themes in both light and dark modes
- Limit Theme Options: Offer a small selection of well-designed themes rather than many similar options
- Theme Consistency: Maintain consistent styling across different themes to avoid UI surprises
Next Steps
Now that you understand how to work with themes in MkSaaS, explore these related topics: