Fonts
Learn how to customize the fonts in your MkSaaS website
This guide covers the font system in your MkSaaS website, how to use the built-in fonts, and how to add and customize your own fonts.
Core Features
The MkSaaS template comes with a carefully selected set of fonts that provide excellent readability and visual appeal. The font system includes:
- Multiple font families for different purposes (sans-serif, serif, monospace)
- Google Fonts integration for easy access to thousands of fonts
- Support for local font files
- CSS variable-based configuration for simple customization
Default Fonts
The template includes several pre-configured fonts that you can use out of the box.
Built-in Font Families
The following font families are available by default:
- Noto Sans: The main sans-serif font used for most text
- Noto Serif: A serif font that can be used for headings or body text
- Noto Sans Mono: A monospace font used for code blocks and technical content
- Bricolage Grotesque: A modern sans-serif font for decorative elements or headings
These fonts are loaded from Google Fonts and are configured in the src/assets/fonts/index.ts
file:
Font Configuration
The fonts are applied to the application in the root layout component:
Notice that fontNotoSans.className
is applied directly, making it the default font for the entire website, while the other fonts are applied as CSS variables that can be used throughout the application.
CSS Variables
The font variables are defined in the global CSS file and can be used in your Tailwind CSS classes:
Adding Custom Fonts
You can add custom fonts to your website in two ways: using Google Fonts or local font files.
Adding Google Fonts
To add a new Google Font:
- Import the font from
next/font/google
- Configure the font with appropriate options
- Export the font variable
For example, to add the Roboto font:
You can explore the Google Fonts website to find the perfect font for your website.
Adding Local Fonts
To use a local font file:
- Download the font files (preferably in WOFF2 format for best performance)
- Place the font files in the
src/assets/fonts
directory - Import
localFont
fromnext/font/local
- Configure the font with appropriate options
For example:
You can download font files from various sources:
- Google Webfonts Helper - Easy tool to download Google Fonts in various formats
- Font Squirrel - Free fonts for commercial use
Using the New Font
After adding a new font, you need to:
- Update the layout component to include the new font variable:
- Optionally, add a CSS variable in
globals.css
:
Changing the Default Font
To change the default font for your entire website:
- Choose which font you want to set as the default
- Update the layout component to use that font's
className
property instead offontNotoSans.className
:
This screenshot shows the default font is now the custom font.
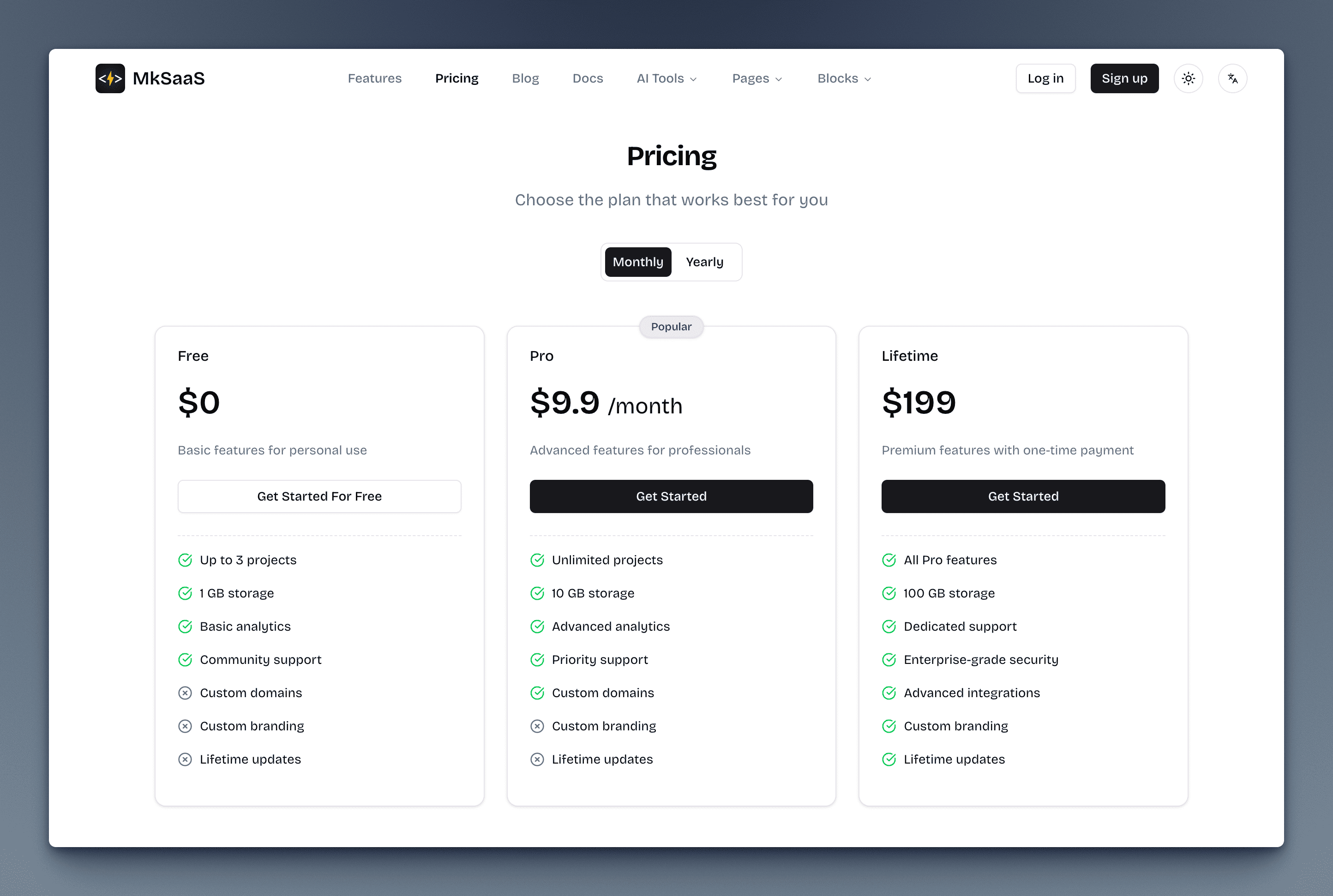
Font Usage Examples
Here are some examples of how to use the different fonts in your components:
Font Subsetting and Performance
Next.js automatically subsets Google Fonts to include only the characters needed for the languages you specify, which improves performance.
The subsets
parameter specifies which character sets to include:
For multilingual websites, you can include additional subsets:
Advanced Font Customization
Font Display Strategy
The display
property controls how the font is loaded and displayed:
Options include:
'swap'
: Show a fallback font until the custom font loads (prevents invisible text)'block'
: Briefly show invisible text, then fallback, then custom font'fallback'
: Similar to swap but with a shorter timeout'optional'
: Let the browser decide whether to use the custom font based on connection
Adjusting Font Weights
You can specify which font weights to include to optimize performance:
This is important for performance as each additional weight increases the amount of font data that needs to be downloaded.
Best Practices
- Limit Font Families: Use no more than 2-3 font families on your website for a cohesive look
- Limit Font Weights: Include only the weights you actually need (typically regular, medium, and bold)
- Use WOFF2 Format: For local fonts, use WOFF2 format for the best compression and performance
- Test Performance: Check your website's performance after adding custom fonts
- Consider Fallbacks: Specify appropriate fallback fonts using Tailwind's font family utilities
- Accessibility: Ensure your chosen fonts are readable for all users, especially for body text
Next Steps
Now that you understand how to work with fonts in MkSaaS, explore these related topics: